Data converter¶
Data converters are required to read or write the byte data available via GInOut in a typed manner, e.g. as an integer.
The following converters are available in Viper.NET:
All converters support the parameter
- TwistBytes
[True|False] With
True
the order of the bytes in the memory is rotated (least significant byte first). WithFalse
the order of the bytes remains unchanged.
Example:
Int16 val = 5;
Byte-Daten [TwistBytes = False]: [0x05][0x00]
Byte-Daten [TwistBytes = True]: [0x00][0x05]
For some converters additional parameters are required.
GenericInputConverter¶
For the GenericInputConverter, in contrast to the other converters, the target type must also be specified. The following target types are available:
Target type |
data length in bytes |
---|---|
Boolean |
1 |
Byte |
1 |
SByte |
1 |
Char |
2 |
Int16 |
2 |
Int32 |
4 |
Int64 |
8 |
UInt16 |
2 |
UInt32 |
4 |
UInt64 |
8 |
Single (=float) |
4 |
Double |
8 |
Decimal |
16 |
Care must be taken to ensure that the expected data length is maintained.
Byte array -> Byte¶
This very simple converter returns or writes the first byte in the byte array.
Byte array -> Byte array¶
The byte data are taken over 1:1. However, it can happen that the data lengths do not match. A distinction must be made between two cases here:
The data area in GInOut is larger than or equal to the byte data to be written.
The data area in GInOut is filled with “MemSetVal”.
The data area in GInOut is smaller than the data to be written:
Invalid, generates an error (The data cannot be written).
Care must therefore be taken to ensure that the data area is sufficiently large.
Additional converter parameters:
- Length
What for again? With “-1” the length of the data area is used.
- MemSetVal
This byte value is used to fill the data area if the data to be written is shorter.
Byte array -> String¶
This converter interprets data as an ASCII-encoded string. For different data lengths, the same rules apply as for Byte array -> Byte array.
Additional converter parameters:
- Length
What for again? With “-1” the length of the data area is used.
- MemSetVal
This byte value is used to fill the data area if the data to be written is shorter.
- TrimCharacters
Listing of all single characters that can represent the end of the string. The characters can be specified either as ASCII characters or in hex notation ([0x31] is the character ‘a’, for example).
Example:
Datenbereich mit Länge 5, Inhalt in HEX: 3132330000
TrimCharacters [0x00]
Der Konverter liefert hier "123"
Byte array -> S7 String¶
This converter interprets data as S7 STRING:
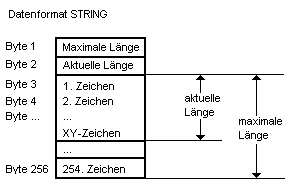
Structure S7 STRING¶
Converter parameters:
- Capacity
Specifies the maximum length of the string. With “-1” the length of the data area is used, which is correct for most use cases.
- MemSetVal
This byte value is used to fill the data area if the data to be written is shorter.
- TrimCharacters
Listing of all single characters that can represent the end of the string. The characters can be specified either as ASCII characters or in hex notation ([0x31] is the character ‘a’, for example).
Example:
Data segment with length=5, content in HEX: 3132330000
TrimCharacters [0x00]
Converter returns "123"
Byte array -> Int16 or Int32¶
These converters have been replaced by the GenericInputConverter and are only required for backward compatibility.
Byte array bits -> Bool, Int16 or Int32¶
With the “Byte array bits” converters, individual bits are read from the data area and a boolean or numerical value is formed. This allows individual bits within a byte to be masked out and the sequence of the bits to be influenced.
Additional converter parameters:
- MapWeightToBitIdx
Comma-separated list of bit indices from the data area that are used for the conversion. The order also forms the weighting.
Example:
Data segment with length=1, value t 0x17. (Binary1: 0b00010111)
MapWeightToBitIdx: 4, 3, 2
Result after conversion: 5 (Bit 4: 1, Bit 3: 0, Bit 2: 1).
Byte array string -> Int16, Int32 or Double¶
The byte array string converters are mainly needed for communication with Waycom Prodel, since this controller provides its data exclusively in ASCII-coded form. A bit in Prodel, for example, always has the value “0x30” (LOW) or “0x31” (HIGH).
The additional converter parameters are analogous to Byte array -> String.
Byte array hex string -> UInt32¶
GenericArrayConverter¶
With this converter arrays of simple value types can be read in. The same data types are supported as with GenericInputConverter. The data length results from the number of elements (Length
), the selected data type, and additional 4 bytes if FixedLength=False
.
In addition to TwistBytes
this converter supports the following parameters:
- MaxCount
Specifies the maximum number of elements in the array.
- FixedCount
[True]: All elements are always valid. [False]: The first 4 bytes in the data area the number indicate how many elements in the array are valid. For the conversion of these bytes the GenericInputConverter for Int32 is used.
GenericBitArrayConverter¶
This converter assigns one of two values to each bit in the data segment, depending on whether the bit is 0 or 1. This method saves space in the data segment if several Boolean information are to be transmitted simultaneously.
- MaxCount
Specifies the maximum number of elements in the array.
- FixedCount
[True]: All elements are always valid. [False]: The first 4 bytes in the data area the number indicate how many elements in the array are valid. For the conversion of these bytes the GenericInputConverter for Int32 is used.
- LowVal
Value in the array if the associated bit is 0.
- HighVal
Value in the array if the associated bit is 1.
StringArrayConverter¶
Analogous to GenericArrayConverter this converter can be used to convert arrays of strings. The strings have a maximum length. For the strings themselves Byte array -> String is used.
- MaxCount
Specifies the maximum number of elements in the array.
- FixedCount
[True]: All elements are always valid. [False]: The first 4 bytes in the data area the number indicate how many elements in the array are valid. For the conversion of these bytes the GenericInputConverter for Int32 is used.
- Length
The maximum length of a string in the array.
- MemSetVal
See also Byte array -> String
- TrimCharacters
See also Byte array -> String